NumPy Library
NumPy 라이브러리
- Numerical Python의 약어이다.
- 고성능 과학 계산용 패키지로 강력한 N-Dimension Array Object(\(\texttt{ndarray}\) Type)로 구성되어 있다.
(범용적 데이터 처리에 용이한 Multiple-Dimensinoal Container)
- 정교한 Broadcasting이 가능하다.
(형태가 다른 Matrix끼리 혹은 다른 타입의 수치들에 대한 연산이 가능하도록 조정하는 것을 "Broadcasting"이라 부른다.)
Python List와 NumPy array의 차이
- NumPy array는 Homogeneous Containter이고, Python List는 Heterogeneous Container이다.
(NumPy array는 단일 타입의 원소만을 허용하며, Python List는 다양한 타입의 원소들을 허용한다.)
- NumPy array는 단일 타입만을 허용함으로써 높은 성능(실행시간 및 메모리 효율성)을 낼 수 있게한 자료구조이고,
Python List는 다양한 타입을 허용함으로써 범용성을 높인 자료구조이다.
Installation (설치)
Installation Command (설치 명령어)
# Installation of NumPy libraries for Python can be done using pip
pip install numpy
# Installation of NumPy libraries for Python can be done using anaconda
conda install numpy
Import (모듈 임포트)
# Recommended import style
import numpy as np
NumPy Array Object (NumPy 배열 객체)
NumPy Array Object | Description |
The N-dimensional array (\(\texttt{numpy.ndarray}\)) |
- NumPy에서는 동일한 타입의 데이터를 담을 수 있는 N차원 배열 객체를 제공한다. - Python List보다 빠르고 메모리 효율적으로 동작한다. |
Scalars | - NumPy에서는 Scientific Computing을 위한, Python Built-in Types보다 정교한 데이터 타입들을 제공한다. - CPython으로 구현되었다. |
Data type objects (\(\texttt{numpy.dtype}\)) |
- NumPy array에 대해, 아래와 같은Meta Data를 제공한다: 1) Type of the data 2) Size of the data 3) Byte order of the data (Little-Endian or Big-Endian) 4) Meta data of structured data type (Field name, Field type, Memory size of each field) 5) Shape and type of sub-array 6) \(\texttt{datetime64}\) and \(\texttt{timedelta64}\) |
Indexing routines | - NumPy에서는 NumPy array(Matrix)에 대해 다양한 Indexing을 수행할 수 있게 하는 함수들을 제공한다. |
Iterating Over Arrays (\(\texttt{numpy.nditer}\)) |
- 하나 이상의 NumPy Array에 대한 Flexible한 Iteration을 제공한다. |
Standard array subclasses | - \(\texttt{ndarray}\)는 상속되어 다양한 형태로 재정의될 수 있다. - NumPy에서는 \(\texttt{ndarray}\)를 상속받은 새로운 객체가 다른 array객체와 원활히 Interaction할 수 있도록 하는 여러 Tool들을 제공한다. |
Masked arrays (\(\texttt{numpy.ma}\)) |
- Missing Entry 혹은 Invalid Entry를 위한 배열로, NumPy에서는 Masking 역할을 수행하는 배열 객체를 제공한다. |
The array interface protocol (Array Protocol) |
- array와 같은 Python Obejct들이 서로의 Data Buffer를 재사용할 수 있게 하는 프로토콜이다. |
Datatimes and Timedeltas (\(\texttt{numpy.datetime64}\)) (\(\texttt{numpy.timedelta64}\)) |
- NumPy에서는 자체적으로 날짜 및 시간에 관련된 기능을 제공한다. - Python Standard Library에 이미 \(\texttt{datetime}\) 이름이 존재하기 때문에, NumPy에서는 \(\texttt{datetime64}\)로 정의되었다. - NumPy에서는 \(\texttt{timedelta64}\)를 통해 날짜 및 시간에 대해 연산을 가능하게 한다. |
NumPy ndarray Obejct (ndarray 객체)
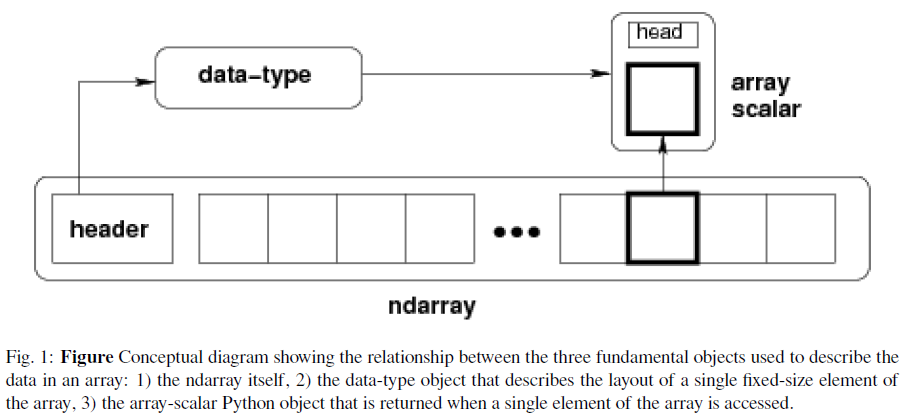
ndarray Constructor
# ndarray Constructor
numpy.ndarray(
shape, # Shape of created array [Tuple of ints]
dtype=float, # Any object that can be interpreted as a numpy data type [Data-type, Optional]
buffer=None, # Used to fill the array with data [Object exposing buffer interface, Optional]
offset=0, # Offset of array data in buffer [int, Optional]
strides=None, # Strides of data in memory [Tuple of ints, Optional]
order=None # Row-major (C-Style) or Column-major(Fortran-Style) order [{'C', 'F'}, Optional]
)
- buffer=None인 경우, Constructor는 shape, dtype, order 매개변수만을 사용하여 ndarray 객체를 생성한다.
- ndarray에서는 __new__() Method가 array 객체의 내부 전체를 Initialization하기 때문에,
__init__() Method의 호출을 필요로 하지 않는다.
Example. ndarray Constructor
# Case: buffer is None
>>> np.ndarray(shape=(2,2), dtype=float, order='F')
array([[0.0e+000, 0.0e+000], # random
[ nan, 2.5e-323]])
# Case: buffer is not None
>>> np.ndarray((2,), buffer=np.array([1,2,3]),
... offset=np.int_().itemsize,
... dtype=int) # offset = 1*itemsize, i.e. skip first element
array([2, 3])
ndarray Attributes
Attribute | Return Type | Description |
T | ndarray | - The transposed array. |
data | buffer | - Python buffer object pointing to the start of the array’s data. |
dtype | dtype object | - Data-type of the array’s elements. |
flags | dict | - Information about the memory layout of the array. |
flat | numpy.flatiter object | - A 1-D iterator over the array. |
imag | ndarray | - The imaginary part of the array. |
real | ndarray | - The real part of the array. |
size | int | - Number of elements in the array. |
itemsize | int | - Length of one array element in bytes. |
nbytes | int | - Total bytes consumed by the elements of the array. |
ndim | int | - Number of array dimensions. |
shape | tuple of ints | - Tuple of array dimensions. |
strides | tuple of ints | - Tuple of bytes to step in each dimension when traversing an array. |
ctypes | ctypes object | - An object to simplify the interaction of the array with the ctypes module. |
base | ndarray | - Base object if memory is from some other object. |
ndarray Methods (Summary)
Method | Description |
all() | - Returns True if all elements evaluate to True. |
any() | - Returns True if any of the elements of a evaluate to True. |
argmax() | - Return indices of the maximum values along the given axis. |
argmin() | - Return indices of the minimum values along the given axis. |
argpartition() | - Returns the indices that would partition this array. |
argsort() | - Returns the indices that would sort this array. |
astype() | - Copy of the array, cast to a specified type. |
byteswap() | - Swap the bytes of the array elements |
choose() | - Use an index array to construct a new array from a set of choices. |
clip() | - Return an array whose values are limited to [min, max]. |
compress() | - Return selected slices of this array along given axis. |
conj() | - Complex-conjugate all elements. |
conjugate() | - Return the complex conjugate, element-wise. |
copy() | - Return a copy of the array. |
cumprod() | - Return the cumulative product of the elements along the given axis. |
cumsum() | - Return the cumulative sum of the elements along the given axis. |
diagonal() | - Return specified diagonals. |
dump() | - Dump a pickle of the array to the specified file. |
dumps() | - Returns the pickle of the array as a string. |
fill() | - Fill the array with a scalar value. |
flatten() | - Return a copy of the array collapsed into one dimension. |
getfield() | - Returns a field of the given array as a certain type. |
item() | - Copy an element of an array to a standard Python scalar and return it. |
itemset() | - Insert scalar into an array (scalar is cast to array’s dtype, if possible) |
max() | - Return the maximum along a given axis. |
mean() | - Returns the average of the array elements along given axis. |
min() | - Return the minimum along a given axis. |
newbyteorder() | - Return the array with the same data viewed with a different byte order. |
nonzero() | - Return the indices of the elements that are non-zero. |
partition() | - Rearranges the elements in the array in such a way that the value of the element in kth position is in the position it would be in a sorted array. |
prod() | - Return the product of the array elements over the given axis |
ptp() | - Peak to peak (maximum - minimum) value along a given axis. |
put() | - Set a.flat[n] = values[n] for all n in indices. |
ravel() | - Return a flattened array. |
repeat() | - Repeat elements of an array. |
reshape() | - Returns an array containing the same data with a new shape. |
resize() | - Change shape and size of array in-place. |
round() | - Return a with each element rounded to the given number of decimals. |
searchsorted() | - Find indices where elements of v should be inserted in a to maintain order. |
setfield() | - Put a value into a specified place in a field defined by a data-type. |
setflags() | - Set array flags WRITEABLE, ALIGNED, WRITEBACKIFCOPY, respectively. |
sort() | - Sort an array in-place. |
squeeze() | - Remove axes of length one from a. |
std() | - Returns the standard deviation of the array elements along given axis. |
sum() | - Return the sum of the array elements over the given axis. |
swapaxes() | - Return a view of the array with axis1 and axis2 interchanged. |
take() | - Return an array formed from the elements of a at the given indices. |
tobytes() | - Construct Python bytes containing the raw data bytes in the array. |
tofile() | - Write array to a file as text or binary (default). |
tolist() | - Return the array as an a.ndim-levels deep nested list of Python scalars. |
tostring() | - A compatibility alias for tobytes, with exactly the same behavior. |
trace() | - Return the sum along diagonals of the array. |
transpose() | - Returns a view of the array with axes transposed. |
var() | - Returns the variance of the array elements, along given axis. |
view() | - New view of array with the same data. |
ndarray Methods (Details, Signatures)
Array Creation Routines (배열 생성 함수)
Array Creation Routines (Summary)
Category | Routine | Description |
Array Creation from Shape or Value |
empty() | Return a new array of given shape and type, without initializing entries. |
empty_like() | Return a new array with the same shape and type as a given array. |
|
eye() | Return a 2-D array with ones on the diagonal and zeros elsewhere. |
|
identify() | Return the identity array. | |
ones() | Return a new array of given shape and type, filled with ones. |
|
ones_like() | Return an array of ones with the same shape and type as a given array. |
|
zeros() | Return a new array of given shape and type, filled with zeros. |
|
zeros_like() | Return an array of zeros with the same shape and type as a given array. |
|
full() | Return a new array of given shape and type, filled with fill_value. |
|
full_like() | Return a full array with the same shape and type as a given array. |
|
Array Creation from Existing Data |
array() | Create an array. |
asarray() | Convert the input to an array. | |
asanyarray() | Convert the input to an ndarray, but pass ndarray subclasses through. |
|
ascontiguousarray() | Return a contiguous array (ndim >= 1) in memory (C order). | |
asmatrix() | Interpret the input as a matrix. | |
copy() | Return an array copy of the given object. | |
frombuffer() | Interpret a buffer as a 1-dimensional array. | |
from_dlpack() | Create a NumPy array from an object implementing the __dlpack__ protocol. |
|
fromfile() | Construct an array from data in a text or binary file. | |
fromfunction() | Construct an array by executing a function over each coordinate. | |
fromiter() | Create a new 1-dimensional array from an iterable object. | |
fromstring() | A new 1-D array initialized from text data in a string. | |
loadtxt() | Load data from a text file. | |
Record Array Creation (numpy.core.records) |
core.records.array() | Construct a record array from a wide-variety of objects. |
core.records.fromarrays() | Create a record array from a (flat) list of arrays | |
core.records.fromrecords() | Create a recarray from a list of records in text form. | |
core.records.fromstring() | Create a record array from binary data | |
core.records.fromfile() | Create an array from binary file data | |
Characters Array Creation (numpy.core.defchararray) |
core.defchararray.array() | Create a chararray. |
core.defchararray.asarray() | Convert the input to a chararray, copying the data only if necessary. |
|
Numerical Ranges |
arange() | Return evenly spaced values within a given interval. |
linspace() | Return evenly spaced numbers over a specified interval. | |
logspace() | Return numbers spaced evenly on a log scale. | |
geomspace() | Return numbers spaced evenly on a log scale (a geometric progression). |
|
meshgrid() | Return coordinate matrices from coordinate vectors. | |
mgrid() | nd_grid instance which returns a dense multi-dimensional ”meshgrid”. |
|
ogrid() | nd_grid instance which returns an open multidimensional ”meshgrid”. |
|
Building Matirx |
diag() | Extract a diagonal or construct a diagonal array. |
diagflat() | Create a two-dimensional array with the flattened input as a diagonal. |
|
tri() | An array with ones at and below the given diagonal and zeros elsewhere. |
|
tril() | Lower triangle of an array. | |
triu() | Upper triangle of an array. | |
vander() | Generate a Vandermonde matrix. | |
Matrix Class |
mat() | Interpret the input as a matrix. |
bmat() | Build a matrix object from a string, nested sequence, or array. |
Array Creation Routines (Details, Signatures)
Array Manipulation Routines (배열 조작 함수)
Array Manipulation Routines (Summary)
Category | Routine | Description |
Basic Operations |
copyto() | Copies values from one array to another, broadcasting as necessary. |
shape() | Return the shape of an array. | |
Changing Array Shape |
reshape() | Gives a new shape to an array without changing its data. |
ravel() | Return a contiguous flattened array. | |
ndarray.flat | A 1-D iterator over the array. | |
ndarray.flatten() | Return a copy of the array collapsed into one dimension. | |
Transpose-Like Operations |
moveaxis() | Move axes of an array to new positions. |
rollaxis() | Roll the specified axis backwards, until it lies in a given position. |
|
swapaxes() | Interchange two axes of an array. | |
ndarray.T | The transposed array. | |
transpose() | Reverse or permute the axes of an array; returns the modified array. |
|
Changing Number of Dimensions |
atleast_1d() | Convert inputs to arrays with at least one dimension. |
atleast_2d() | View inputs as arrays with at least two dimensions. | |
atleast_3d() | atleast_3d(*arys) View inputs as arrays with at least three dimensions. | |
broadcast | broadcast Produce an object that mimics broadcasting. | |
broadcast_to() | broadcast_to(array, shape[, subok]) Broadcast an array to a new shape. | |
broadcast_arrays() | broadcast_arrays(*args[, subok]) Broadcast any number of arrays against each other. | |
expand_dims() | expand_dims(a, axis) Expand the shape of an array. | |
squeeze() | squeeze(a[, axis]) Remove axes of length one from a. | |
Changing kind of Array |
asarray() | asarray(a[, dtype, order, like]) Convert the input to an array. |
asanyarray() | Convert the input to an ndarray, but pass ndarray subclasses through. |
|
asmatrix() | asmatrix(data[, dtype]) Interpret the input as a matrix. | |
asfarray() | asfarray(a[, dtype]) Return an array converted to a float type. | |
asfortranarray() | Return an array (ndim >= 1) laid out in Fortran order in memory. |
|
ascontiguousarray() | Return a contiguous array (ndim >= 1) in memory (C order). | |
asarray_chkfinite() | asarray_chkfinite(a[, dtype, order]) Convert the input to an array, checking for NaNs or Infs. | |
require() | Return an ndarray of the provided type that satisfies requirements. | |
Joining Arrays |
concatenate() | Join a sequence of arrays along an existing axis. |
stack() | Join a sequence of arrays along a new axis. | |
block() | Assemble an nd-array from nested lists of blocks. | |
vstack() | Stack arrays in sequence vertically (row wise). | |
hstack() | Stack arrays in sequence horizontally (column wise). | |
dstack() | Stack arrays in sequence depth wise (along third axis). | |
column_stack() | Stack 1-D arrays as columns into a 2-D array. | |
row_stack() | Stack arrays in sequence vertically (row wise). | |
Splitting Arrays |
split() | Split an array into multiple sub-arrays as views into ary. |
array_split() | Split an array into multiple sub-arrays. | |
dsplit() | Split array into multiple sub-arrays along the 3rd axis (depth). |
|
hsplit() | Split an array into multiple sub-arrays horizontally (column-wise). |
|
vsplit() | Split an array into multiple sub-arrays vertically (rowwise). | |
Tiling Arrays |
tile() | Construct an array by repeating A the number of times given by reps. |
repeat() | Repeat elements of an array. | |
Adding and Removing Elements |
delete() | Return a new array with sub-arrays along an axis deleted. |
insert() | Insert values along the given axis before the given indices. | |
append() | Append values to the end of an array. | |
resize() | Return a new array with the specified shape. | |
trim_zeros() | Trim the leading and/or trailing zeros from a 1-D array or sequence. |
|
unique() | Find the unique elements of an array. | |
Rearranging Elements |
flip() | Reverse the order of elements in an array along the given axis. |
fliplr() | Reverse the order of elements along axis 1 (left/right). | |
flipud() | Reverse the order of elements along axis 0 (up/down). | |
reshape() | Gives a new shape to an array without changing its data. | |
roll() | Roll array elements along a given axis. | |
rot90() | Rotate an array by 90 degrees in the plane specified by axes. |
Reference: "NumPy Documentation", NumPy.org, 2022.08.15 검색, URL.
Reference: "NumPy documentation", Runebook.dev, 2022.08.16 검색, URL.
NumPy Library
NumPy 라이브러리
- Numerical Python의 약어이다.
- 고성능 과학 계산용 패키지로 강력한 N-Dimension Array Object(\(\texttt{ndarray}\) Type)로 구성되어 있다.
(범용적 데이터 처리에 용이한 Multiple-Dimensinoal Container)
- 정교한 Broadcasting이 가능하다.
(형태가 다른 Matrix끼리 혹은 다른 타입의 수치들에 대한 연산이 가능하도록 조정하는 것을 "Broadcasting"이라 부른다.)
Python List와 NumPy array의 차이
- NumPy array는 Homogeneous Containter이고, Python List는 Heterogeneous Container이다.
(NumPy array는 단일 타입의 원소만을 허용하며, Python List는 다양한 타입의 원소들을 허용한다.)
- NumPy array는 단일 타입만을 허용함으로써 높은 성능(실행시간 및 메모리 효율성)을 낼 수 있게한 자료구조이고,
Python List는 다양한 타입을 허용함으로써 범용성을 높인 자료구조이다.
Installation (설치)
Installation Command (설치 명령어)
# Installation of NumPy libraries for Python can be done using pip
pip install numpy
# Installation of NumPy libraries for Python can be done using anaconda
conda install numpy
Import (모듈 임포트)
# Recommended import style
import numpy as np
NumPy Array Object (NumPy 배열 객체)
NumPy Array Object | Description |
The N-dimensional array (\(\texttt{numpy.ndarray}\)) |
- NumPy에서는 동일한 타입의 데이터를 담을 수 있는 N차원 배열 객체를 제공한다. - Python List보다 빠르고 메모리 효율적으로 동작한다. |
Scalars | - NumPy에서는 Scientific Computing을 위한, Python Built-in Types보다 정교한 데이터 타입들을 제공한다. - CPython으로 구현되었다. |
Data type objects (\(\texttt{numpy.dtype}\)) |
- NumPy array에 대해, 아래와 같은Meta Data를 제공한다: 1) Type of the data 2) Size of the data 3) Byte order of the data (Little-Endian or Big-Endian) 4) Meta data of structured data type (Field name, Field type, Memory size of each field) 5) Shape and type of sub-array 6) \(\texttt{datetime64}\) and \(\texttt{timedelta64}\) |
Indexing routines | - NumPy에서는 NumPy array(Matrix)에 대해 다양한 Indexing을 수행할 수 있게 하는 함수들을 제공한다. |
Iterating Over Arrays (\(\texttt{numpy.nditer}\)) |
- 하나 이상의 NumPy Array에 대한 Flexible한 Iteration을 제공한다. |
Standard array subclasses | - \(\texttt{ndarray}\)는 상속되어 다양한 형태로 재정의될 수 있다. - NumPy에서는 \(\texttt{ndarray}\)를 상속받은 새로운 객체가 다른 array객체와 원활히 Interaction할 수 있도록 하는 여러 Tool들을 제공한다. |
Masked arrays (\(\texttt{numpy.ma}\)) |
- Missing Entry 혹은 Invalid Entry를 위한 배열로, NumPy에서는 Masking 역할을 수행하는 배열 객체를 제공한다. |
The array interface protocol (Array Protocol) |
- array와 같은 Python Obejct들이 서로의 Data Buffer를 재사용할 수 있게 하는 프로토콜이다. |
Datatimes and Timedeltas (\(\texttt{numpy.datetime64}\)) (\(\texttt{numpy.timedelta64}\)) |
- NumPy에서는 자체적으로 날짜 및 시간에 관련된 기능을 제공한다. - Python Standard Library에 이미 \(\texttt{datetime}\) 이름이 존재하기 때문에, NumPy에서는 \(\texttt{datetime64}\)로 정의되었다. - NumPy에서는 \(\texttt{timedelta64}\)를 통해 날짜 및 시간에 대해 연산을 가능하게 한다. |
NumPy ndarray Obejct (ndarray 객체)
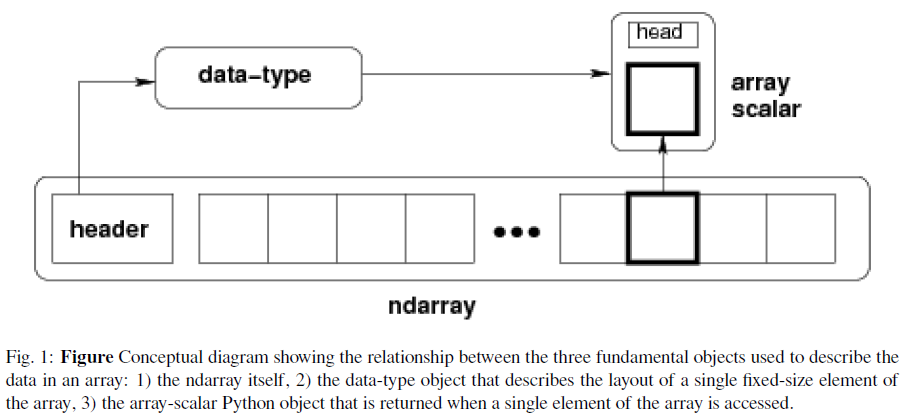
ndarray Constructor
# ndarray Constructor
numpy.ndarray(
shape, # Shape of created array [Tuple of ints]
dtype=float, # Any object that can be interpreted as a numpy data type [Data-type, Optional]
buffer=None, # Used to fill the array with data [Object exposing buffer interface, Optional]
offset=0, # Offset of array data in buffer [int, Optional]
strides=None, # Strides of data in memory [Tuple of ints, Optional]
order=None # Row-major (C-Style) or Column-major(Fortran-Style) order [{'C', 'F'}, Optional]
)
- buffer=None인 경우, Constructor는 shape, dtype, order 매개변수만을 사용하여 ndarray 객체를 생성한다.
- ndarray에서는 __new__() Method가 array 객체의 내부 전체를 Initialization하기 때문에,
__init__() Method의 호출을 필요로 하지 않는다.
Example. ndarray Constructor
# Case: buffer is None
>>> np.ndarray(shape=(2,2), dtype=float, order='F')
array([[0.0e+000, 0.0e+000], # random
[ nan, 2.5e-323]])
# Case: buffer is not None
>>> np.ndarray((2,), buffer=np.array([1,2,3]),
... offset=np.int_().itemsize,
... dtype=int) # offset = 1*itemsize, i.e. skip first element
array([2, 3])
ndarray Attributes
Attribute | Return Type | Description |
T | ndarray | - The transposed array. |
data | buffer | - Python buffer object pointing to the start of the array’s data. |
dtype | dtype object | - Data-type of the array’s elements. |
flags | dict | - Information about the memory layout of the array. |
flat | numpy.flatiter object | - A 1-D iterator over the array. |
imag | ndarray | - The imaginary part of the array. |
real | ndarray | - The real part of the array. |
size | int | - Number of elements in the array. |
itemsize | int | - Length of one array element in bytes. |
nbytes | int | - Total bytes consumed by the elements of the array. |
ndim | int | - Number of array dimensions. |
shape | tuple of ints | - Tuple of array dimensions. |
strides | tuple of ints | - Tuple of bytes to step in each dimension when traversing an array. |
ctypes | ctypes object | - An object to simplify the interaction of the array with the ctypes module. |
base | ndarray | - Base object if memory is from some other object. |
ndarray Methods (Summary)
Method | Description |
all() | - Returns True if all elements evaluate to True. |
any() | - Returns True if any of the elements of a evaluate to True. |
argmax() | - Return indices of the maximum values along the given axis. |
argmin() | - Return indices of the minimum values along the given axis. |
argpartition() | - Returns the indices that would partition this array. |
argsort() | - Returns the indices that would sort this array. |
astype() | - Copy of the array, cast to a specified type. |
byteswap() | - Swap the bytes of the array elements |
choose() | - Use an index array to construct a new array from a set of choices. |
clip() | - Return an array whose values are limited to [min, max]. |
compress() | - Return selected slices of this array along given axis. |
conj() | - Complex-conjugate all elements. |
conjugate() | - Return the complex conjugate, element-wise. |
copy() | - Return a copy of the array. |
cumprod() | - Return the cumulative product of the elements along the given axis. |
cumsum() | - Return the cumulative sum of the elements along the given axis. |
diagonal() | - Return specified diagonals. |
dump() | - Dump a pickle of the array to the specified file. |
dumps() | - Returns the pickle of the array as a string. |
fill() | - Fill the array with a scalar value. |
flatten() | - Return a copy of the array collapsed into one dimension. |
getfield() | - Returns a field of the given array as a certain type. |
item() | - Copy an element of an array to a standard Python scalar and return it. |
itemset() | - Insert scalar into an array (scalar is cast to array’s dtype, if possible) |
max() | - Return the maximum along a given axis. |
mean() | - Returns the average of the array elements along given axis. |
min() | - Return the minimum along a given axis. |
newbyteorder() | - Return the array with the same data viewed with a different byte order. |
nonzero() | - Return the indices of the elements that are non-zero. |
partition() | - Rearranges the elements in the array in such a way that the value of the element in kth position is in the position it would be in a sorted array. |
prod() | - Return the product of the array elements over the given axis |
ptp() | - Peak to peak (maximum - minimum) value along a given axis. |
put() | - Set a.flat[n] = values[n] for all n in indices. |
ravel() | - Return a flattened array. |
repeat() | - Repeat elements of an array. |
reshape() | - Returns an array containing the same data with a new shape. |
resize() | - Change shape and size of array in-place. |
round() | - Return a with each element rounded to the given number of decimals. |
searchsorted() | - Find indices where elements of v should be inserted in a to maintain order. |
setfield() | - Put a value into a specified place in a field defined by a data-type. |
setflags() | - Set array flags WRITEABLE, ALIGNED, WRITEBACKIFCOPY, respectively. |
sort() | - Sort an array in-place. |
squeeze() | - Remove axes of length one from a. |
std() | - Returns the standard deviation of the array elements along given axis. |
sum() | - Return the sum of the array elements over the given axis. |
swapaxes() | - Return a view of the array with axis1 and axis2 interchanged. |
take() | - Return an array formed from the elements of a at the given indices. |
tobytes() | - Construct Python bytes containing the raw data bytes in the array. |
tofile() | - Write array to a file as text or binary (default). |
tolist() | - Return the array as an a.ndim-levels deep nested list of Python scalars. |
tostring() | - A compatibility alias for tobytes, with exactly the same behavior. |
trace() | - Return the sum along diagonals of the array. |
transpose() | - Returns a view of the array with axes transposed. |
var() | - Returns the variance of the array elements, along given axis. |
view() | - New view of array with the same data. |
ndarray Methods (Details, Signatures)
Array Creation Routines (배열 생성 함수)
Array Creation Routines (Summary)
Category | Routine | Description |
Array Creation from Shape or Value |
empty() | Return a new array of given shape and type, without initializing entries. |
empty_like() | Return a new array with the same shape and type as a given array. |
|
eye() | Return a 2-D array with ones on the diagonal and zeros elsewhere. |
|
identify() | Return the identity array. | |
ones() | Return a new array of given shape and type, filled with ones. |
|
ones_like() | Return an array of ones with the same shape and type as a given array. |
|
zeros() | Return a new array of given shape and type, filled with zeros. |
|
zeros_like() | Return an array of zeros with the same shape and type as a given array. |
|
full() | Return a new array of given shape and type, filled with fill_value. |
|
full_like() | Return a full array with the same shape and type as a given array. |
|
Array Creation from Existing Data |
array() | Create an array. |
asarray() | Convert the input to an array. | |
asanyarray() | Convert the input to an ndarray, but pass ndarray subclasses through. |
|
ascontiguousarray() | Return a contiguous array (ndim >= 1) in memory (C order). | |
asmatrix() | Interpret the input as a matrix. | |
copy() | Return an array copy of the given object. | |
frombuffer() | Interpret a buffer as a 1-dimensional array. | |
from_dlpack() | Create a NumPy array from an object implementing the __dlpack__ protocol. |
|
fromfile() | Construct an array from data in a text or binary file. | |
fromfunction() | Construct an array by executing a function over each coordinate. | |
fromiter() | Create a new 1-dimensional array from an iterable object. | |
fromstring() | A new 1-D array initialized from text data in a string. | |
loadtxt() | Load data from a text file. | |
Record Array Creation (numpy.core.records) |
core.records.array() | Construct a record array from a wide-variety of objects. |
core.records.fromarrays() | Create a record array from a (flat) list of arrays | |
core.records.fromrecords() | Create a recarray from a list of records in text form. | |
core.records.fromstring() | Create a record array from binary data | |
core.records.fromfile() | Create an array from binary file data | |
Characters Array Creation (numpy.core.defchararray) |
core.defchararray.array() | Create a chararray. |
core.defchararray.asarray() | Convert the input to a chararray, copying the data only if necessary. |
|
Numerical Ranges |
arange() | Return evenly spaced values within a given interval. |
linspace() | Return evenly spaced numbers over a specified interval. | |
logspace() | Return numbers spaced evenly on a log scale. | |
geomspace() | Return numbers spaced evenly on a log scale (a geometric progression). |
|
meshgrid() | Return coordinate matrices from coordinate vectors. | |
mgrid() | nd_grid instance which returns a dense multi-dimensional ”meshgrid”. |
|
ogrid() | nd_grid instance which returns an open multidimensional ”meshgrid”. |
|
Building Matirx |
diag() | Extract a diagonal or construct a diagonal array. |
diagflat() | Create a two-dimensional array with the flattened input as a diagonal. |
|
tri() | An array with ones at and below the given diagonal and zeros elsewhere. |
|
tril() | Lower triangle of an array. | |
triu() | Upper triangle of an array. | |
vander() | Generate a Vandermonde matrix. | |
Matrix Class |
mat() | Interpret the input as a matrix. |
bmat() | Build a matrix object from a string, nested sequence, or array. |
Array Creation Routines (Details, Signatures)
Array Manipulation Routines (배열 조작 함수)
Array Manipulation Routines (Summary)
Category | Routine | Description |
Basic Operations |
copyto() | Copies values from one array to another, broadcasting as necessary. |
shape() | Return the shape of an array. | |
Changing Array Shape |
reshape() | Gives a new shape to an array without changing its data. |
ravel() | Return a contiguous flattened array. | |
ndarray.flat | A 1-D iterator over the array. | |
ndarray.flatten() | Return a copy of the array collapsed into one dimension. | |
Transpose-Like Operations |
moveaxis() | Move axes of an array to new positions. |
rollaxis() | Roll the specified axis backwards, until it lies in a given position. |
|
swapaxes() | Interchange two axes of an array. | |
ndarray.T | The transposed array. | |
transpose() | Reverse or permute the axes of an array; returns the modified array. |
|
Changing Number of Dimensions |
atleast_1d() | Convert inputs to arrays with at least one dimension. |
atleast_2d() | View inputs as arrays with at least two dimensions. | |
atleast_3d() | atleast_3d(*arys) View inputs as arrays with at least three dimensions. | |
broadcast | broadcast Produce an object that mimics broadcasting. | |
broadcast_to() | broadcast_to(array, shape[, subok]) Broadcast an array to a new shape. | |
broadcast_arrays() | broadcast_arrays(*args[, subok]) Broadcast any number of arrays against each other. | |
expand_dims() | expand_dims(a, axis) Expand the shape of an array. | |
squeeze() | squeeze(a[, axis]) Remove axes of length one from a. | |
Changing kind of Array |
asarray() | asarray(a[, dtype, order, like]) Convert the input to an array. |
asanyarray() | Convert the input to an ndarray, but pass ndarray subclasses through. |
|
asmatrix() | asmatrix(data[, dtype]) Interpret the input as a matrix. | |
asfarray() | asfarray(a[, dtype]) Return an array converted to a float type. | |
asfortranarray() | Return an array (ndim >= 1) laid out in Fortran order in memory. |
|
ascontiguousarray() | Return a contiguous array (ndim >= 1) in memory (C order). | |
asarray_chkfinite() | asarray_chkfinite(a[, dtype, order]) Convert the input to an array, checking for NaNs or Infs. | |
require() | Return an ndarray of the provided type that satisfies requirements. | |
Joining Arrays |
concatenate() | Join a sequence of arrays along an existing axis. |
stack() | Join a sequence of arrays along a new axis. | |
block() | Assemble an nd-array from nested lists of blocks. | |
vstack() | Stack arrays in sequence vertically (row wise). | |
hstack() | Stack arrays in sequence horizontally (column wise). | |
dstack() | Stack arrays in sequence depth wise (along third axis). | |
column_stack() | Stack 1-D arrays as columns into a 2-D array. | |
row_stack() | Stack arrays in sequence vertically (row wise). | |
Splitting Arrays |
split() | Split an array into multiple sub-arrays as views into ary. |
array_split() | Split an array into multiple sub-arrays. | |
dsplit() | Split array into multiple sub-arrays along the 3rd axis (depth). |
|
hsplit() | Split an array into multiple sub-arrays horizontally (column-wise). |
|
vsplit() | Split an array into multiple sub-arrays vertically (rowwise). | |
Tiling Arrays |
tile() | Construct an array by repeating A the number of times given by reps. |
repeat() | Repeat elements of an array. | |
Adding and Removing Elements |
delete() | Return a new array with sub-arrays along an axis deleted. |
insert() | Insert values along the given axis before the given indices. | |
append() | Append values to the end of an array. | |
resize() | Return a new array with the specified shape. | |
trim_zeros() | Trim the leading and/or trailing zeros from a 1-D array or sequence. |
|
unique() | Find the unique elements of an array. | |
Rearranging Elements |
flip() | Reverse the order of elements in an array along the given axis. |
fliplr() | Reverse the order of elements along axis 1 (left/right). | |
flipud() | Reverse the order of elements along axis 0 (up/down). | |
reshape() | Gives a new shape to an array without changing its data. | |
roll() | Roll array elements along a given axis. | |
rot90() | Rotate an array by 90 degrees in the plane specified by axes. |
Reference: "NumPy Documentation", NumPy.org, 2022.08.15 검색, URL.
Reference: "NumPy documentation", Runebook.dev, 2022.08.16 검색, URL.